This article shows how to use the Entity Framework - Microsoft's recommended data access technology for new applications. For demo purposes, this article describes how to create a simple Windows Forms application using TX Text Control .NET for Windows Forms and the MailMerge component. The MailMerge component is available in all .NET based TX Text Control products and can be used in ASP.NET, WPF and Windows Forms.
MailMerge can be directly used with data objects generated by the Entity Framework using the MergeObjects method.
Requirements
Visual Studio 2012 or better is required to complete this tutorial.
Creating the Application
-
Open Visual Studio and select New -> Project from the File main menu.
-
Choose Windows in the left hand Template tree view and Windows Forms Application as the project template.
-
Enter TXEntityFrameworkSample as the name and confirm with OK.
Install the Entity Framework NuGet Package
-
In Solution Explorer, right-click on the TXEntityFrameworkSample project and select Manage NuGet Packages....
-
In the Manage NuGet Packages dialog, select the Online tab and choose the EntityFramework package.
-
Confirm with Install and follow the installation instructions. Hide the dialog by clicking Close.
Adding TX Text Control and MailMerge to the Window
-
Double-click Form1.cs in the Solution Explorer to open the form's designer. Find the TX Text Control 22.0 toolbox tab that has been created automatically. All usable TX Text Control controls or components are listed in this tab. Choose the TextControl icon and draw it on the form.
-
Now, click on the Smart Tag in the upper right corner of TextControl. In the Wizards group, click on Add a Button Bar, Add a Status Bar, Add a Ruler Bar and Add a Vertical Ruler. Finally, click on Arrange Controls Automatically. The controls are now connected and docked to fill the container.
-
Find the MailMerge component in the Toolbox and drag and drop an instance onto the form. Click on the Smart Tag and choose Connect to Text Component.
Define a Model - Database First
For this tutorial, we will create a new database for demo purposes. The EF designer will reverse engineer your model from this database.
-
Choose Server Explorer from the View main menu.
-
Right click on Data Connections -> Add Connection… to open the Add Connection dialog.
-
Select Microsoft SQL Server as the data source and connect to either LocalDb ((localdb)\v11.0) or SQL Express (.\SQLEXPRESS), depending on which you have installed, and enter Products as the database name:
-
Confirm with OK. You will be asked, if you want to create a new database. Confirm with Yes.
-
The newly created database will appear in Server Explorer. Right-click on it and select New Query.
-
Copy the following SQL into the query, then right-click on the query and select Execute.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters. Learn more about bidirectional Unicode charactersCREATE TABLE [dbo].[Categories] ( [CategoryId] [int] NOT NULL IDENTITY, [Name] [nvarchar](max), CONSTRAINT [PK_dbo.Categories] PRIMARY KEY ([CategoryId]) ) CREATE TABLE [dbo].[Products] ( [ProductId] [int] NOT NULL IDENTITY, [Name] [nvarchar](max), [CategoryId] [int] NOT NULL, CONSTRAINT [PK_dbo.Products] PRIMARY KEY ([ProductId]) ) CREATE INDEX [IX_CategoryId] ON [dbo].[Products]([CategoryId]) ALTER TABLE [dbo].[Products] ADD CONSTRAINT [FK_dbo.Products_dbo.Categories_CategoryId] FOREIGN KEY ([CategoryId]) REFERENCES [dbo].[Categories] ([CategoryId]) ON DELETE CASCADE SET IDENTITY_INSERT [dbo].[Categories] ON INSERT INTO [dbo].[Categories] ([CategoryId], [Name]) VALUES (1, N'Category 1') INSERT INTO [dbo].[Categories] ([CategoryId], [Name]) VALUES (2, N'Category 2') INSERT INTO [dbo].[Categories] ([CategoryId], [Name]) VALUES (3, N'Category 3') SET IDENTITY_INSERT [dbo].[Categories] OFF SET IDENTITY_INSERT [dbo].[Products] ON INSERT INTO [dbo].[Products] ([ProductId], [Name], [CategoryId]) VALUES (2, N'Product 1', 1) INSERT INTO [dbo].[Products] ([ProductId], [Name], [CategoryId]) VALUES (3, N'Product 2', 2) INSERT INTO [dbo].[Products] ([ProductId], [Name], [CategoryId]) VALUES (4, N'Product 3', 1) SET IDENTITY_INSERT [dbo].[Products] OFF
Create Model with the EF Designer
-
Select Add New Item... from the Project main menu.
-
Select Data from the left menu and ADO.NET Entity Data Model as the template.
-
Enter ProductModel as the name and confirm with OK.
-
In the opened dialog Entity Data Model Wizard, select Generate from Database and click Next.
-
Select the connection to the created database, enter ProductContext as the name of the connection string and click Next.
-
Check the checkbox next to Tables to import all tables and confirm with Finish.
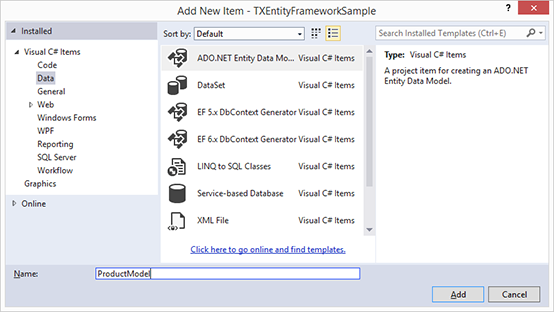
Merging Templates Using MailMerge
-
Download the sample template template.tx.
-
Select TXEntityFrameworkSample in the Solution Explorer and choose Add Existing Item... from the Project main menu. Browse for the downloaded template.tx file, select it and confirm with Add.
-
Select the newly added file template.tx in the Solution Explorer and set the Copy to Output Directory property to Copy always.
-
Select Form1.cs in the Solution Explorer and choose Code from the View main menu. Replace the existing code with the following code:
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters. Learn more about bidirectional Unicode charactersusing System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace TXEntityFrameworkSample { public partial class Form1 : Form { public Form1() { InitializeComponent(); } protected override void OnLoad(EventArgs e) { base.OnLoad(e); ProductContext _context = new ProductContext(); textControl1.Load("template.tx", TXTextControl.StreamType.InternalUnicodeFormat); mailMerge1.MergeObjects(_context.Products); } } }
Compile and start the application.
TX Text Control's reporting engine MailMerge is using the template that is loaded into the connected TextControl instance in order to merge it with data from the generated DbSet object.
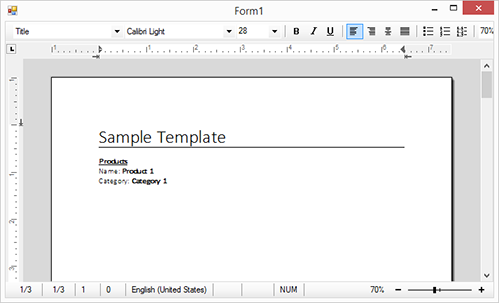