TX Text Control .NET for Windows Forms 14.0 introduced the ApplicationField class to manipulate MS Word standard fields like MergeField, Date or NumPages. All switches and parameters of these fields are represented by the Parameters property which gets or sets a string array of the available values.
In order to create or edit such fields, you need to know the structure of these standard fields. In version 15.0, we introduced the FieldAdapter classes for the most commonly used fields:
- MergeField
- DateField
- IfField
- IncludeText
- FormCheckbox
- FormDropDown
- FormText
These adapter classes can be used to create or manipulate these MS Word compatible fields without learning the specific switches and possible parameters. All settings can be easily accessed using the available properties.
Let's have a look at a typical MergeField:
{MERGEFIELD company \* Upper \b "Company:" \* MERGEFORMAT}
The following illustration shows this field in MS Word with a description of each parameter:
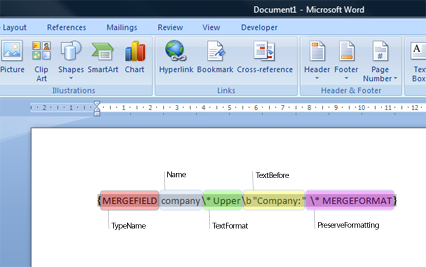
By accesssing this field using the ApplicationFields, the Parameter property would return this string array:
{ "company", "\* Upper", "\b \"Company\"", "\* MERGEFORMAT" }
How does the FieldAdapter comes into play?
As the name implies, FieldAdapter is an adapter for the ApplicationFields. It can be only used in combination with these fields. To manipulate a MergeField, the MergeField adapter should be used. To create a new instance of the MergeField, the specific ApplicationField can be passed in the constructor:
foreach (TXTextControl.ApplicationField field in textControl1.ApplicationFields) { if(field.TypeName == "MERGEFIELD") { TXTextControl.DocumentServer.Fields.MergeField mergeField = new TXTextControl.DocumentServer.Fields.MergeField(field); mergeField.Name = "companyname"; } }
In the above code, we check the TypeName of the field. If it is a MergeField, a new instance of the adapter class is created and the ApplicationField is passed in the constructor. After that, we have access to the specific MergeField properties like the Name. You can use the FieldAdapter to insert new fields as well:
TXTextControl.DocumentServer.Fields.MergeField mergeField = new TXTextControl.DocumentServer.Fields.MergeField(); textControl1.ApplicationFields.Add(mergeField.ApplicationField);
Summary: Using the FieldAdapters, you can easily handle the most commonly used fields of MS Word. You don't need to care about parsing the parameters on your own. You get a full set of properties for each available setting.