AutoCorrect can be very annoying on a smartphone - we all know this. But if you are used to it and then start typing on a computer keyboard, you are missing this time saving feature.

TX Spell .NET provides a very powerful spell checking and suggestion engine that can be used to implement AutoCorrect with a few lines of code:
string sLastStoredWordText; | |
private void textControl1_KeyPress(object sender, KeyPressEventArgs e) | |
{ | |
// delimiter array | |
char[] cDelimiters = new char[] { ',','.','\r','\t',' ' }; | |
// check, if a delimiter has been pressed and if | |
// misspelled words have been found | |
if (!cDelimiters.Contains(e.KeyChar) || | |
textControl1.MisspelledWords == null || | |
textControl1.MisspelledWords.Count == 0) | |
return; | |
int iTextPosition = textControl1.InputPosition.TextPosition; | |
// find a misspelled word at the input position | |
MisspelledWord mwLastWord = | |
textControl1.MisspelledWords.GetItem(iTextPosition); | |
// if the found word is not at the input position | |
// store the last word and return | |
if (mwLastWord.Start + mwLastWord.Length != iTextPosition + 1) | |
{ | |
sLastStoredWordText = mwLastWord.Text; return; | |
} | |
// if the found word is the last word, store it and return | |
// this prevents an auto replacement for intentionally wrong words | |
if (mwLastWord.Text == sLastStoredWordText) | |
{ | |
sLastStoredWordText = mwLastWord.Text; return; | |
} | |
// store the last word | |
sLastStoredWordText = mwLastWord.Text; | |
// create the suggestions, we only need the first | |
txSpellChecker1.CreateSuggestions(mwLastWord.Text, 1); | |
// if suggestions are found, replace the word with the suggestion | |
if (txSpellChecker1.Suggestions.Count > 0) | |
{ | |
e.Handled = true; | |
string sSuggestion = txSpellChecker1.Suggestions[0].Text + " "; | |
textControl1.MisspelledWords.Remove(mwLastWord, sSuggestion); | |
textControl1.Select(mwLastWord.Start + sSuggestion.Length, 0); | |
} | |
} |
Based on the analysis of thousands of test documents, the expected suggestion is ranked at position 1 or 2 in more than 97% of all cases. The algorithm includes the measurement of the distance between the keys on the currently used keyboard. Many different factors are evaluated and rated to create the accurate list of suggestions.
Essentially, the KeyPress event is used to trap keystrokes when a delimiter such as a space, a '.' or a ',' is typed in. Afer that, the misspelled word at the current input position is checked and replaced with the first suggestion of the TX Spell .NET suggestion engine.
This implementation checks also, if you intentionally typed a word incorrect. The second time you "correct" the correction, AutoCorrect is not applied to the same word again.
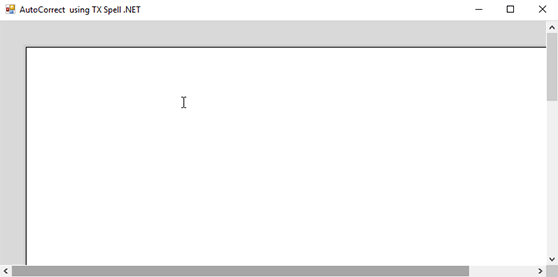
Happy coding!