This sample project shows how to create and insert a watermark image on all pages dynamically. Image objects have the Name property to store additional string information with an image. This property is used to tag the inserted watermark images in order to find them in the ImageCollection.
In a first step, all watermark images are removed. In order to remove the specific images, all tagged images are collected in a List and finally removed from the ImageCollection:
private void RemoveWatermarkImages(TXTextControl.TextControl textControl) | |
{ | |
// List of images to be deleted | |
List<TXTextControl.Image> lImagesToRemove = | |
new List<TXTextControl.Image>(); | |
// loop through all images and check for the Name | |
foreach (TXTextControl.Image img in textControl1.Images) | |
{ | |
// add watermark images to the List | |
if (img.Name == "Watermark") | |
lImagesToRemove.Add(img); | |
} | |
// remove all tagged watermark images | |
foreach(TXTextControl.Image img in lImagesToRemove) | |
{ | |
textControl1.Images.Remove(img); | |
} | |
} |
After that, on each page, a new watermark image is inserted at a fixed position, vertically and horizontally centered. The Name property is set to "Watermark" and the image won't be sizable and moveable.
private void InsertWatermarkImages() | |
{ | |
// remove all existing watermarks | |
RemoveWatermarkImages(textControl1); | |
foreach (TXTextControl.Page page in textControl1.GetPages()) | |
{ | |
// create a new watermark image | |
Bitmap bmp = CreateDraftImage(); | |
// create a new TXTextControl.Image object and mark | |
// as watermark | |
TXTextControl.Image img = new TXTextControl.Image(bmp); | |
img.Name = "Watermark"; | |
img.Sizeable = false; | |
img.Moveable = false; | |
// calculate the location to center the image | |
Point pImageLocation = new Point( | |
(page.Bounds.Width / 2) - (PixelToTwips(bmp.Size.Width)) / 2, | |
(page.Bounds.Height / 2) - (PixelToTwips(bmp.Size.Height)) / 2); | |
// add the image to the page | |
textControl1.Images.Add( | |
img, | |
page.Number, | |
pImageLocation, | |
TXTextControl.ImageInsertionMode.BelowTheText); | |
} | |
} |
On the TextControl Changed event, a simple condition checks whether the current number of pages has been increased. If yes, a new watermark is inserted:
private int iNumberOfPages = 1; | |
// update the watermarks if the number of pages are different | |
private void textControl1_Changed(object sender, EventArgs e) | |
{ | |
if (textControl1.Pages > iNumberOfPages) | |
{ | |
InsertWatermarkImages(); | |
iNumberOfPages = textControl1.Pages; | |
} | |
} |
To complete the code description: The method CreateDraftImage returns a Bitmap with the gray text "DRAFT" that is used as a watermark image.
// creates a new "DRAFT" sample image | |
private Bitmap CreateDraftImage() | |
{ | |
string sText = "DRAFT"; | |
Bitmap destination = new Bitmap(400, 400); | |
using (Graphics g = Graphics.FromImage(destination)) | |
{ | |
GraphicsUnit units = GraphicsUnit.Pixel; | |
g.Clear(Color.White); | |
StringFormat stringFormat = new StringFormat(); | |
stringFormat.Alignment = StringAlignment.Center; | |
stringFormat.LineAlignment = StringAlignment.Center; | |
g.DrawString(sText, new Font( | |
"Arial", | |
90, | |
FontStyle.Bold, | |
GraphicsUnit.Pixel), | |
new SolidBrush( | |
Color.FromArgb(64, Color.Black)), | |
destination.GetBounds(ref units), | |
stringFormat); | |
} | |
return destination; | |
} |
The following screenshot shows the watermarks in the TX Text Control .NET for Windows Forms:
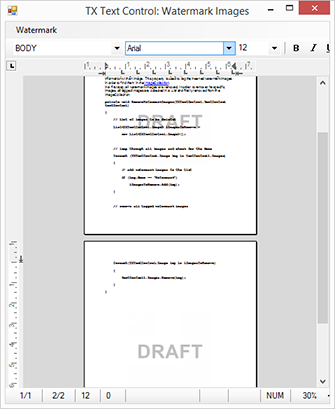
Download the sample from GitHub and test it on your own.