In documents and reports, often sentences or an appendix are added only under specific conditions. The Text Control reporting engine MailMerge provides the concept of INCLUDETEXT fields to include separate, formatted documents at a specific input position in the template. The included documents can be loaded from a physical file in various supported formats such as DOCX, DOC and RTF or formatted text can be injected by handling the IncludeTextMerging event.
The insertion can be also canceled in the same event by returning a blank document. This article shows a best practice how to include sub-templates under specific conditions controlled by a field in the data source.
The Template
In the template, all possible INCLUDETEXT fields are added at the desired locations. In this sample, these fields are inserted adjacent to each other to avoid empty lines in the final document when an INCLUDETEXT field is conditionally removed.
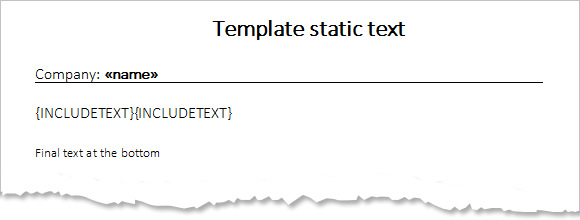
The INCLUDETEXT fields get the filename of the sub-templates: phrase1.docx and phrase2.docx.
The Data Source
This sample uses an XML file as the data source with 3 data rows. Each data row contains a field for the included sub-templates (in this case: phrase1.docx and phrase2.docx). As a convention, the value 1 means that the sub-template should be added and 0 means, it should be omitted.
<journal> <report> <name>Alpha Beta Group, LLC</name> <phrase1.docx>1</phrase1.docx> <phrase2.docx>1</phrase2.docx> </report> <report> <name>Gamma Consulting Corporation</name> <phrase1.docx>0</phrase1.docx> <phrase2.docx>1</phrase2.docx> </report> <report> <name>Delta Fitness Company</name> <phrase1.docx>1</phrase1.docx> <phrase2.docx>0</phrase2.docx> </report> </journal>
Event Handling
To control the conditional sub-templates, 2 events must be handled: DataRowMerged to count the currently merged data row and IncludeTextMerging to check whether the sub-template should be inserted or not.
int iCurrentDataRow = 0; private void mailMerge1_DataRowMerged(object sender, TXTextControl.DocumentServer.MailMerge.DataRowMergedEventArgs e) { iCurrentDataRow = e.DataRowNumber + 1; } private void mailMerge1_IncludeTextMerging(object sender, TXTextControl.DocumentServer.MailMerge.IncludeTextMergingEventArgs e) { byte[] data; // create blank document as byte array using(TXTextControl.ServerTextControl tx = new TXTextControl.ServerTextControl()) { tx.Create(); tx.Save(out data, TXTextControl.BinaryStreamType.InternalUnicodeFormat); } // remove the include text field in case the data contains a "0" if (ds.Tables[0].Rows[iCurrentDataRow][e.Filename].ToString() == "0") e.IncludeTextDocument = data; }
In the IncludeTextMerging event, the data field that matches the name of the INCLUDETEXT field is verified to include or omit the insertion. The code in the events is generalized as it doesn't contain any hard coded field names and the data source itself is used to control the insertion.
The results of the above sample are 3 documents where both sub-templates are included in the first document, the first is omitted in the second document and only the first phrase is inserted in the third document:
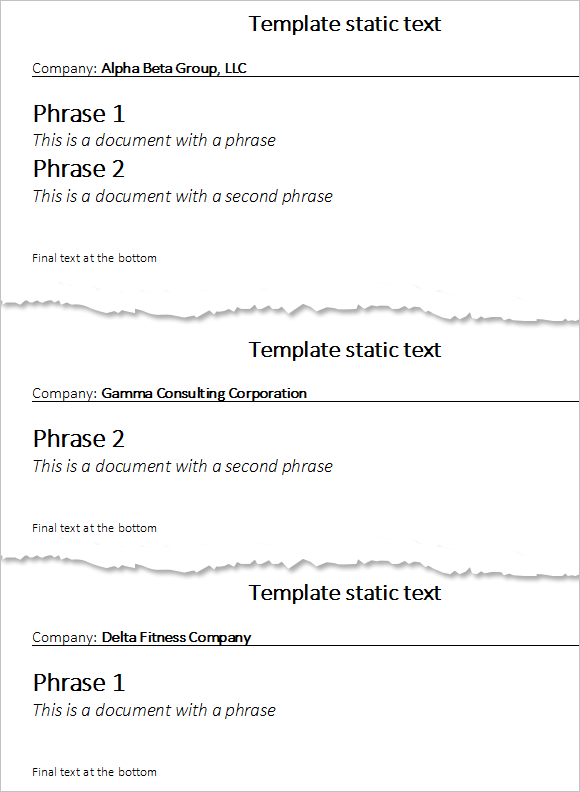
Download the sample from GitHub and test it on your own.