A conditional text block is a range of formatted text that is rendered, if a specified condition is fulfilled. This text block can consist of anything that is supported by TX Text Control: Formatted text, tables, images, other merge fields or sub-templates with IncludeText fields.
The advantage of conditional text blocks is that these blocks are part of the same template and not externalized to sub-templates. Template designers can design all possible content in the same template.
The data source of our sample application has a very simple structure:
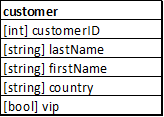
Based on 2 fields, conditional content should be rendered or not. If the field country equals "United States" in the current data record, a defined table should be rendered. Additionally, a specific sentence should be rendered in case the condition is fulfilled. In case the field vip is "True", a header should be inserted into the final report.
The sample data source has 3 records:
<customer> <customerID>1</customerID> <lastName>Jackson</lastName> <firstName>Paul</firstName> <country>United States</country> <vip>True</vip> </customer> <customer> <customerID>2</customerID> <lastName>Murphey</lastName> <firstName>Peter</firstName> <country>Germany</country> <vip>True</vip> </customer> <customer> <customerID>3</customerID> <lastName>Hollister</lastName> <firstName>Phyllis</firstName> <country>Italy</country> <vip>False</vip> </customer>
The following illustration shows 3 different results created based on the same template with conditional text blocks.
In order to insert a conditional block, 2 IF-fields are required with a specific format.
{ IF "country" <> "United States" "%%StartIF%%" "" }
{ IF "country" <> "United States" "%%EndIF%%" "" }
The content between these 2 IF-fields is removed, if the condition is true. In our case: The content between these 2 fields is rendered, if country equals "United States".
The following screenshot shows the template that is used in this sample. The green-marked areas define conditional text blocks with various conditions.
Technically, the IF-fields render unique placeholders when a specific condition is fulfilled. The text between these markers is removed in the DataRowMerged event:
private void mailMerge1_DataRowMerged(object sender, TXTextControl.DocumentServer.MailMerge.DataRowMergedEventArgs e) { byte[] data; string sPlaceholderStartIF = "%%StartIF%%"; string sPlaceholderEndIF = "%%EndIF%%"; using (TXTextControl.ServerTextControl tx = new TXTextControl.ServerTextControl()) { tx.Create(); tx.Load(e.MergedRow, TXTextControl.BinaryStreamType.InternalUnicodeFormat); foreach (TXTextControl.IFormattedText part in tx.TextParts) { do { int start = part.Find(sPlaceholderStartIF, 0, TXTextControl.FindOptions.NoMessageBox); int end = part.Find(sPlaceholderEndIF, start, TXTextControl.FindOptions.NoMessageBox); if (start == -1) continue; part.Selection.Start = start; part.Selection.Length = end - start + sPlaceholderEndIF.Length; part.Selection.Text = ""; } while (part.Find(sPlaceholderStartIF, 0, TXTextControl.FindOptions.NoMessageBox) != -1); } tx.Save(out data, TXTextControl.BinaryStreamType.InternalUnicodeFormat); } e.MergedRow = data; }
Those IF-blocks can be placed everywhere in the template including main text, headers, footers and text frames. Try this on your own and download the sample Visual Studio 2012 project. At least, a TX Text Control .NET for Windows Forms trial version is required.