Companies and organizations in any industry understand the value of electronic signatures embedded in applications and internal workflows to reduce paper, speed up transactions and to provide a better user experience for signing processes.
Integrated Electronic Signatures
Using document processing libraries from Text Control, modern, secure and reliable electronic signatures can be easily integrated into any application using ASP.NET Core, Angular or desktop applications.
Instead of using third-party services such as DocuSign or Adobe Sign, many companies would like to embed electronic signature processes in internal enterprise applications or commercial products.
Electronic? Digital? What is the Difference?
TX Text Control supports electronic signatures and digital signatures. But what is the difference?
- Electronic Signatures
An electronic signature is a simple signature representation that is drawn or uploaded as an image and placed on top of a document. TX Text Control supports two different methods to implement this feature:
- Signature Fields
Pre-defined signature fields can be inserted into documents that can be electronically signed using a signature soft pad.
- Signature Annotations
Instead of signing pre-defined fields, signature images can be acquired in order to insert the signature image as an annotation object to the document.
- Signature Fields
- Digital Signatures
A digital signature combines the electronic signature with a digital certificate that identifies the signer. To ensure that a document has not been altered by a third-party and to verify that the author is the expected person, documents can be digitally signed.
Easy Integration
The following code snipped shows how easy it is to sign an added signature field with an SVG image as the visual signature representation. The created digital signature is associated with the signature field before it is exported as an Adobe PDF document.
public static void SignFields() { | |
using (ServerTextControl tx = new ServerTextControl()) { | |
tx.Create(); | |
// create a signature field | |
SignatureField signatureField = new SignatureField( | |
new System.Drawing.Size(2000, 2000), "txsign", 10); | |
// set image representation | |
signatureField.Image = new SignatureImage("signature.svg", 0); | |
// insert the field | |
tx.SignatureFields.Add(signatureField, -1); | |
// create a digital signature (for each field, if required) | |
DigitalSignature digitalSignature = new DigitalSignature( | |
new System.Security.Cryptography.X509Certificates.X509Certificate2( | |
"textcontrolself.pfx", "123"), null, "txsign"); | |
// apply the signatures to the SaveSettings | |
SaveSettings saveSettings = new SaveSettings() { | |
SignatureFields = new DigitalSignature[] { digitalSignature } | |
}; | |
// export to PDF | |
tx.Save("signed_fields.pdf", StreamType.AdobePDF, saveSettings); | |
} | |
} |
Learn More
Requesting signatures is a common application using the TX Text Control document viewer available for ASP.NET, ASP.NET Core and Angular. This article shows a typical workflow how to prepare documents for a signature acquisition.
What is a Digital Signature?
A digital signature use the concept of hashing - a mathematical function that converts a block of data into a fixed-size string. The result of this function is always identical for this block of data, if the data block has not been altered.
This hash function is used on almost all elements of a document and is then stored inside the document.
The two main advantages of a digital signature are:
- Authentication
A digital certificate allows the exact identification of the author of a signed document.
- Integrity
A digital signature can be used to validate whether the contents of the signed document has been altered after the signing process.
When signing a document, the digital signature generates a unique hash from the document data and a private key. When verified, the data and a public key is used to generate the same hash. If they match, the document is not altered and the signature is valid.
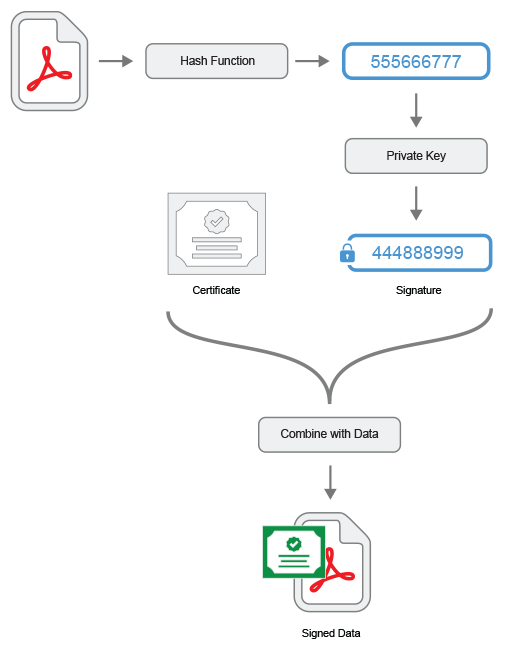
Signing process: Combining data and a private key
Developer Libraries from Text Control
Using the developer libraries from Text Control, the complete electronic signing process can be embedded into any web application with components for ASP.NET, ASP.NET Core with .NET and .NET 7 support, Angular and other JavaScript platforms. Integrate ready-to-use and tested components to deliver intuitive, reliable and safe signature processes to your users.
Learn More
Using TX Text Control, there are two ways of signing PDF documents: The whole document and individual signature fields. This article shows how to implement both ways in a .NET 6 command line application.