The Text Control Reporting engine MailMerge can populate fields automatically during the merge process. These fields can be also combined with MS Word compatible form fields such as checkboxes.
This sample project shows how to display checkbox fields in the HTML5 based Web.TextControl and how to handle the Javascript event TextFieldClicked.
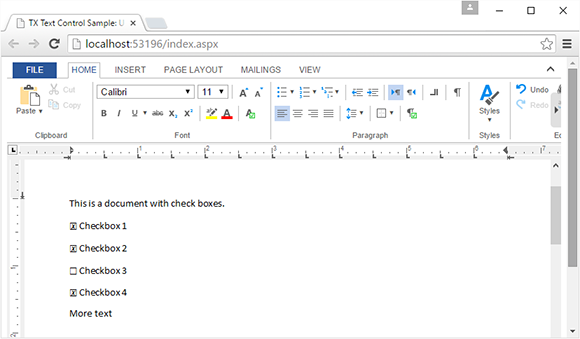
The following method loads the document into a temporary ServerTextControl to loop through all ApplicationFields. When FormCheckBoxes are found, a Checked or Unchecked Unicode character is set based on the checked status of the field.
/******************************************************** | |
* ProcessCheckboxFields method | |
* desc: Sets the Unicode characters for all | |
* checkbox fields | |
* parameter: document - the document in the internal | |
* Text Control format | |
********************************************************/ | |
private byte[] ProcessCheckboxFields(byte[] document) | |
{ | |
// create a new temporary ServerTextControl | |
using (TXTextControl.ServerTextControl tx = | |
new TXTextControl.ServerTextControl()) | |
{ | |
// load the document | |
tx.Create(); | |
tx.Load(document, | |
TXTextControl.BinaryStreamType.InternalUnicodeFormat); | |
// loop through all ApplicationFields | |
foreach (IFormattedText textPart in tx.TextParts) | |
{ | |
foreach (ApplicationField field in textPart.ApplicationFields) | |
{ | |
if ((field.TypeName != "FORMCHECKBOX")) | |
return null; | |
// create a new adapter field | |
FormCheckBox checkboxField = new FormCheckBox(field); | |
// select the field to change the font name | |
textPart.Selection.Start = checkboxField.Start - 1; | |
textPart.Selection.Length = checkboxField.Length; | |
textPart.Selection.FontName = "Arial Unicode MS"; | |
// set the text (state) | |
checkboxField.Text = | |
checkboxField.Checked == true ? CHECKED : UNCHECKED; | |
} | |
} | |
tx.Save(out document, BinaryStreamType.InternalUnicodeFormat); | |
return document; | |
} | |
} |
In the client-side Javascript, the TX Text Control event TextFieldClicked is used to update the AJAX UpdatePanel with the clicked field name as a parameter:
<script type="text/javascript"> | |
// attach the 'textFieldClicked' event | |
TXTextControl.addEventListener("textFieldClicked", function (e) { | |
fieldClicked(e.fieldName, e.fieldType, e.typeName); | |
}); | |
// do an AJAX postback on the UpdatePanel | |
function fieldClicked(fieldName, fieldType, typeName) { | |
__doPostBack('<%= UpdatePanel1.ClientID %>', fieldName); | |
} | |
</script> |
In the code-behind, the AJAX call is processed and based on the argument, the clicked checkbox field is toggled.
protected void Page_Load(object sender, EventArgs e) | |
{ | |
// handle the AJAX postback | |
string eventTarget = | |
Convert.ToString(Request.Params.Get("__EVENTTARGET")); | |
string eventArgument = | |
Convert.ToString(Request.Params.Get("__EVENTARGUMENT")); | |
// if the event argument is set, toggle the checkbox | |
if (eventArgument != null) | |
{ | |
ToggleCheckBox(eventArgument); | |
} | |
} |
Try the complete sample by cloning the repository from GitHub. Happy coding!