Recently, we released a new version of the DocumentViewer that supports custom signature handling by routing the signature data and/or signed document to a given, custom endpoint in your ASP.NET web application.
Server-Side Processing
This is useful, if the signed document is stored server-side or needs to be further processed in your application. The sample project discussed in this article forwards the signed document and the associated data to a given URL. The following sequence diagram shows this workflow:
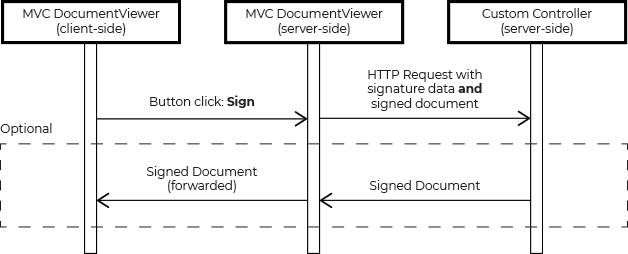
Sequence diagram: Sending signature data and signed document
Read more
Learn more about all supported workflows in this detailed article about the new functionality:
Define the Redirect URL
The following HTML helper code shows how to add the DocumentViewer to the view and to set the RedirectUrlAfterSignature to the custom Home/HandleSignature controller in the same application.
@Html.TXTextControl().DocumentViewer(settings => { | |
settings.DocumentPath = "App_Data/nda.tx"; | |
settings.Dock = DocumentViewerSettings.DockStyle.Fill; | |
settings.SignatureSettings = new SignatureSettings() { | |
RedirectUrlAfterSignature = this.Url.Action( | |
"HandleSignature", | |
"Home", | |
new { securityToken = "1234" }, | |
Context.Request.Scheme, | |
null), | |
ShowSignatureBar = true, | |
SignerName = "Tim Typer", | |
UniqueId = "1234-1234-1234-1234" | |
}; | |
}).Render() |
Additionally, a query string parameter is given to show that additional, custom data can be forwarded to the request. In this sample, a simple securityToken string is forwarded to "validate" the request. In a real-world application, this could be any other data or a valid OAuth access token.
The custom controller HttpPost method HandleSignature is called by the DocumentViewer after a document has been signed successfully:
[HttpPost] | |
public IActionResult HandleSignature([FromBody] SignatureData data, string securityToken) | |
{ | |
ReturnObject returnObject = new ReturnObject(); | |
if (securityToken != "123") | |
{ | |
return Ok(returnObject); | |
} | |
// here, you have access to the signed document and other meta data | |
var test = data.SignedDocument.Document; | |
returnObject.Success = true; | |
returnObject.Id = data.SignedDocument.UniqueId; | |
return Ok(returnObject); | |
} | |
public class ReturnObject | |
{ | |
public string Id { get; set; } | |
public bool Success { get; set; } = false; | |
} |
The forwarded SignatureData object contains meta data about the signature and the signed document:
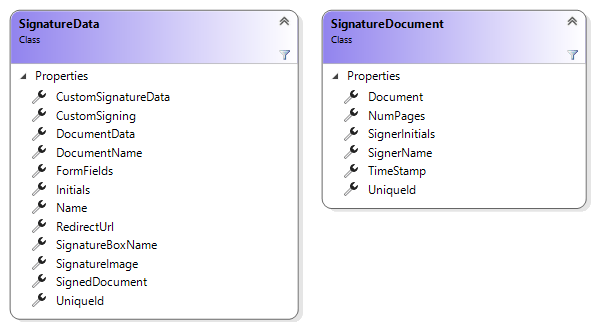
The securityToken is checked for "validity". If valid, a return object is created with information that should be passed back to client-side JavaScript. This is not required, but useful to provide a feedback to users. Implicitly, to be flexible, the DocumentViewer will always return a string and your custom endpoint should return a 200 status code in all cases - even if your custom method is failing. From the signing perspective, the action is fulfilled and successful. Therefore, in this demo, a ReturnObject is returned with a Success property.
Client-Side Callback
On client-side, the setSubmitCallback method is used to attach a method that is called after a successful roundtrip to your custom controller method:
// attach the callback | |
window.addEventListener("documentViewerLoaded", function () { | |
TXDocumentViewer.signatures.setSubmitCallback(handleSignedDocument); | |
}); | |
function handleSignedDocument(data) { | |
var returnObject = JSON.parse(data); | |
if (returnObject.success === true) | |
alert("Thanks for signing!"); | |
else | |
alert("Signing failed!"); | |
} |
As the return value is always a string, it can be parsed into an object and directly accessed.
Test this on your own and download the sample application from our GitHub account.