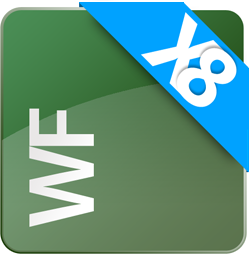
In various applications, it might be neccessary to estimate whether a string with a specific font and size would fit into a fixed space like a table cell. In GDI+, you can use MeasureString to measure a specified string when drawn with a specified Font.
In TX Text Control, this is different. TX Text Control has it's own text rendering based on many different factors including a printer driver. This rendering is known as WYSIWYG and makes a rich edit control a good and usable control.
But how to get the extension of a string without adding the text to the TextControl instance?
Since version X8, the non-visible ServerTextControl can be used in Windows Forms and WPF applications as well. This component can be used to get the length of a text. The following simple function shows how to do that:
///<summary> ///Returns the length of a specific string in Twips. ///</summary> public int MeasureTextControlString(Selection Selection, string FormattingPrinter) { int iLength; using (ServerTextControl tx = new ServerTextControl()) { tx.Create(); tx.PageSize.Width = 10000; tx.FormattingPrinter = FormattingPrinter; foreach (PropertyInfo property in Selection.GetType().GetProperties()) { if (property.GetValue(Selection, null).ToString() == "") continue; property.SetValue(tx.Selection, property.GetValue(Selection, null), null); } tx.SelectAll(); return iLength = tx.TextChars[tx.Selection.Length].Bounds.Right - tx.TextChars[tx.Selection.Start + 1].Bounds.Left; } }
It is pretty straightforward. A Selection object is copied and applied to the new ServerTextControl instance. The width of the text is calculated using the TextChar bounds of the first and last character in the Selection. The following code shows how to call this function with a new Selection object:
Selection newSelection = new Selection(); newSelection.Text = "Hello dWorld"; newSelection.FontSize = 600; newSelection.FontName = "Wingdings"; int length = MeasureTextControlString(newSelection, textControl1.FormattingPrinter);
You can specify different properties in the Selection object that are used to calculate the exact width.