Background images can be easily added to pages in TX Text Control by placing the images behind the text. Thanks to flexible methods and insertion mode options, you can simply loop through all pages in order to insert an image at a fixed position on the current page behind the text.
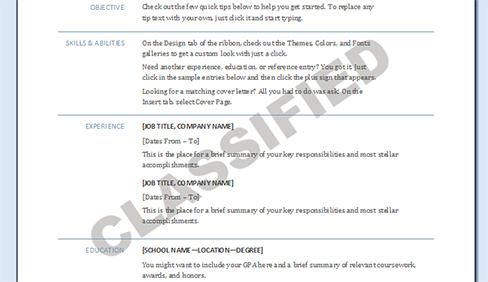
In order to center the image horizontally, the image width must be subtracted from the page width divided by 2 to get the x value for the location. The vertical value is calculated analogically with the horizontal value.
// factor to convert 1/100 inch to twips int dpix = (int)(1440 / textControl1.CreateGraphics().DpiX); // loop through all pages foreach (TXTextControl.Page page in textControl1.GetPages()) { System.Drawing.Image img = Image.FromFile("bgimage.png"); // calculate the position to center the image on the current page int xLocation = (int)(((textControl1.Sections[page.Section].Format.PageSize.Width - img.Size.Width) * dpix) / 2); int yLocation = (int)(((textControl1.Sections[page.Section].Format.PageSize.Height - img.Size.Height) * dpix) / 2); TXTextControl.Image TXimg = new TXTextControl.Image(img); // add the image to the page textControl1.Images.Add( TXimg, page.Number, new Point(xLocation, yLocation), TXTextControl.ImageInsertionMode.FixedOnPage | TXTextControl.ImageInsertionMode.BelowTheText); }