When merging templates with data, a JSON object contains merge data for all merge fields and merge blocks in the document. Using the language specific wrappers, the JSON object is created automatically and you don't need to know the exact expected JSON structure. For example, the .NET wrapper accepts an object as data which is then transformed to the required JSON data implicitly.
Consider the following .NET class:
public class Invoice | |
{ | |
public string name { get; set; } | |
public string invoice_no { get; set; } | |
} |
Now, an instance of that object is created and passed to the MergeData property of the MergeBody object:
// create dummy data | |
Invoice invoice = new Invoice(); | |
invoice.name = "Text Control, LLC"; | |
invoice.invoice_no = "Test_R667663"; | |
// create a new MergeBody object | |
MergeBody body = new MergeBody(); | |
body.MergeData = invoice; |
In the MergeDocument method, the MergeBody object is simply passed as a parameter:
// merge the document | |
List<byte[]> results = rc.MergeDocument(body, templateName, ReturnFormat.PDF); |
Internally, the wrapper is creating the expected JSON format of the complete MergeBody object:
{ | |
"mergeData": { | |
"name": "Text Control, LLC", | |
"invoice_no": "Test_R667663" | |
}, | |
"template": null, | |
"mergeSettings": null | |
} |
The sample template, that gets copied into your template storage when creating a trial account, contains top level merge fields and a merge block that contains separate merge fields:
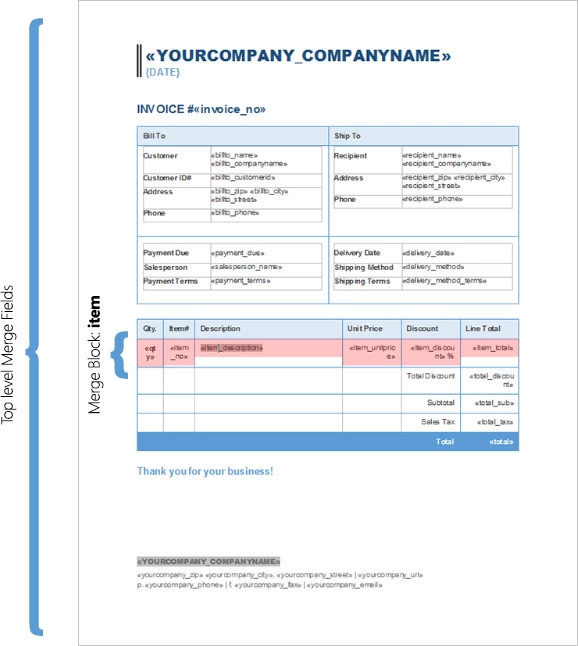
Top level merge fields are all fields in a template that are not part of a merge block. The complete template is repeated based on the number of records (array entries) in the merge data. Merge fields encapsulated in a merge block are repeated based on the number of merge block data rows in the JSON array. Additionally, merge blocks can be nested in unlimited levels. The following JSON data contains 1 top level record:
[ | |
{ | |
"yourcompany_companyname": "Text Control, LLC", | |
"yourcompany_zip": "28226", | |
"item": [ | |
{ | |
"qty": "1", | |
"item_no": "1", | |
"item_description": "Item description 1", | |
"item_unitprice": "2663", | |
"item_discount": "20", | |
"item_total": "2130.40" | |
}, | |
{ | |
"qty": "1", | |
"item_no": "2", | |
"item_description": "Item description 2", | |
"item_unitprice": "5543", | |
"item_discount": "0", | |
"item_total": "5543" | |
} | |
], | |
"total_discount": "532.60", | |
"total_sub": "7673.4", | |
"total_tax": "537.138", | |
"total": "8210.538" | |
} | |
] |
The data is part of an JSON array. The array contains 1 top level entry and this entry has two items in the merge block item. The following sample JSON structure contains 2 top level array entries that produces 2 resulting documents:
[ | |
{ | |
"yourcompany_companyname": "Text Control, LLC", | |
"yourcompany_zip": "28226" | |
}, | |
{ | |
"yourcompany_companyname": "Text Control GmbH", | |
"yourcompany_zip": "28217" | |
} | |
] |