The printing approach in WPF is fundamentally different from printing in Windows Forms. In Windows Forms, you have a PrintDocument and events for each page that can be used for interaction.
In WPF, the PrintTicket class enables you to configure the printer's features such as Collation, CopyCount and PageOrder. The PrintQueue class manages the printers and the print job itself.
Important: Even though the classes PrintQueue and PrintTicket are embedded in the same namespace System.Printing, the PrintQueue class is part of the assembly System.Printing.dll and the PrintTicket class is available through the ReachFramework.dll assembly. Make sure that you are adding both dependencies to your references.
TX Text Control .NET for WPF implements a very easy way to print without any settings to the default printer. This method is easy and works without additional settings:
textControl1.Print(string docName, true);
In order to adjust all printer and print job settings such as the collation, the number of copies and the various page selection options of the printer dialog, these settings must be handled separately. The following code opens a new PrintDialog with all page selection options enabled. The MinPage and MaxPage must be defined based on the existing pages in TextControl.
The user settings are available through the PrintTicket and PrintQueue properties of the PrintDialog class. The PageRangeSelection returns the selected page selection options. Based on these settings, a new PageRange object (customRange) is created and passed to the Print method of TextControl.
PrintDialog dlg = new PrintDialog(); dlg.MinPage = 1; dlg.MaxPage = (uint)textControl1.Pages; dlg.CurrentPageEnabled = true; dlg.SelectedPagesEnabled = true; dlg.UserPageRangeEnabled = true; PageRange customRange = new PageRange(); if (dlg.ShowDialog() == true) { switch (dlg.PageRangeSelection) { case PageRangeSelection.AllPages: // print all pages customRange = new PageRange((int)dlg.MinPage, (int)dlg.MaxPage); break; case PageRangeSelection.CurrentPage: // use the page at the current input position customRange = new PageRange(textControl1.InputPosition.Page); break; case PageRangeSelection.UserPages: // user selection customRange = dlg.PageRange; break; case PageRangeSelection.SelectedPages: // get the start and end page of the current selection int iEndPage = textControl1.InputPosition.Page; textControl1.Selection.Length = 0; int iStartPage = textControl1.InputPosition.Page; customRange = new PageRange(iStartPage, iEndPage); break; } textControl1.Print( "Doc", dlg.PrintQueue.FullName, customRange, (int)dlg.PrintTicket.CopyCount, (Collation)dlg.PrintTicket.Collation);
The following screenshot shows the print dialog and the various available settings:
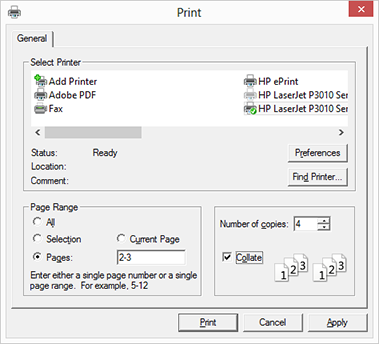
The following table shows the printer dialog options and the appropriate properties:
Print dialog setting | Property |
Page Range: All | PageRangeSelection.AllPages |
Page Range: Selection | PageRangeSelection.SelectedPages |
Page Range: Current Page | PageRangeSelection.CurrentPage |
Page Range: Pages | PageRangeSelection.UserPages, PageRange |
Number of copies | PrintTicket.CopyCount |
Collate | PrintTicket.Collation |
Select(ed) Printer | PrintQueue.FullName |